Loading... # Promise是什么? Promise是一个解决回调地狱的方案 # 什么是回调地狱 函数嵌套,导致可读性差,举个简单的🌰 ``` var sayhello = function (text, callback) { setTimeout(function () { console.log(new Date().getTime(), text); callback(); }, 1000); } sayhello("1.hello", function () { sayhello("2.hello", function () { sayhello("3.hello", function () { setTimeout(function () { console.log(new Date().getTime(), "4.goodbye"); }, 1000); }); }); }); ``` 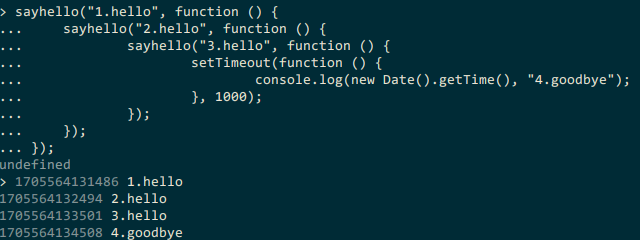 # 示例 ## Promise 举个简单的🌰 ```js console.log('start') new Promise((resolve,reject)=>{ setTimeout(()=>{ console.log('ok') resolve(1) },1000) }).then(res=>console.info('resolve: ',res)) console.log('end') ``` 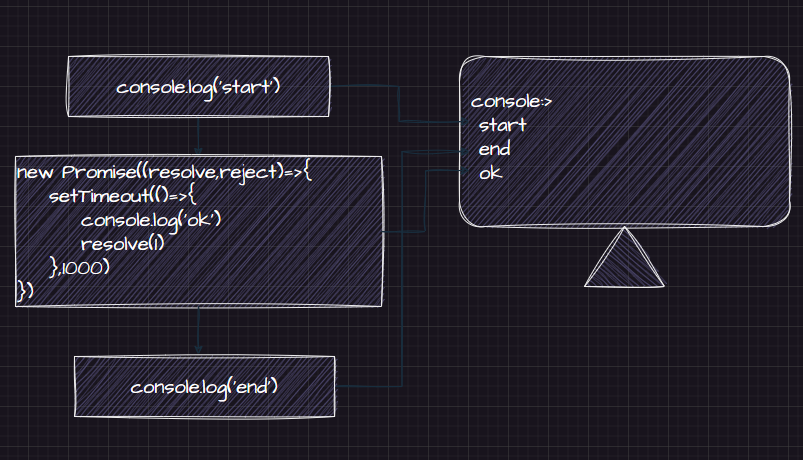 根据上面的demo,可以看到promise相当于启动了一个线程,并没有阻塞,继续执行下面的`console.log('end')`,想要获取Promise中结果,可以使用`.then`方法 ## 同步和异步 在使用Promise之前`async`和`await`应该并不陌生了,举个简单的🌰 ```js function a(){ return new Promise((resolve,reject)=>{ setTimeout(()=>{ console.log(new Date().getTime()) resolve(1) },1000) }) } async function b(){ console.log("res->",await a()); console.log("res->",await a()); console.log("res->",await a()); console.log("res->",await a()); console.log("res->",await a()); } b() ``` 以上示例可以看出来,`await`的结果就是resolve的值,原本`setTimeout`和`Promise`并不会阻塞代码运行,但是在这里就被`await`阻塞了 © 允许规范转载 打赏 赞赏作者 支付宝微信 赞 如果觉得我的文章对你有用,请随意赞赏